How to pass data between iOS 9 views in Swift 2.0
Hey everyone! I’m DJ Spiess and in this video we’re going to talk about how to send data between view controllers using Swift 2.0. The code in this video uses Swift 2.0 on iOS 9. It may or may not work on other versions. Swift is a moving target, but hopefully with Swift 2.0, the dust is starting to settle.
What we’re going to do for this app is to have the user select a color on a second screen. On the main screen we’ll display the color the user selected. On the secondary screen the user can select between red, green, or blue. We’ll also let the user know what color was selected before.
The data passed from the first screen to the second screen is what color was previously selected. We’ll pass that data directly. The data passed from the second screen to the first screen is the color the user selected. We’ll pass it back using delegation. It’s a simple app, but the app we’re creating demonstrates how data is moved in both directions.
Here’s what we have. We start with two view controllers in our storyboard, both embedded in a navigation controller. The source code for this app is available on GitHub if you want to follow along. On the start view we have a subview that’s displaying the color using the background color property. That’s the currently selected color. And we have a button that takes us to the DestinationView. The DestinationView has three buttons, one for three selectable colors. We also have a label to display what color was selected before.
We’ll segue from the StartViewController when the user presses the button. prepareForSegue is the method called before we transition to the next view. We’ll control click to add a segue from the button to the destination view. We’ll just use Show as the segue type, and name the identifier as “pickColorSegue”. That gets us to the destination view. The DestinationView needs to display what was selected before, so we need to add code to pass the value of the previously selected color.
To pass information to the DestinationView, we call prepareForSegue. This method allows us to pass information from the current view to the next. The segue object holds a reference to the view we are segue-ing to. The property is destinationViewController.
It would be nice if we could set the label value directly, but the label outlet is not hooked up when we call prepareForSegue. We need to add a property on the DestinationView to hold the value until we can update the label text. We’ll then use that value in the viewDidLoad method on the DestinationViewController to set the label.
*That’s pretty easy. It gets a bit trickier to send data back. To return data from a modal dialog, or if you’re popping views like we need to with a NavigationController, we need to use the delegate pattern
The basics of the delegate pattern is we call another object to perform the action we need. We delegate the action, and the class we delegate to performs the action. In our case, instead of the DestinationView setting values on the the StartViewController, we’ll delegate that action to the delegate class. The reason we do it this way is because the DestinationView should have no knowledge of the StartView. This way we could use the DestinationView in other places in our application. If that wasn’t clear, let me know in the comments below.
Here’s the fun part. We create a protocol with one method that communicates any information we need. In our case, we’ll create a protocol with one method called setColor(). This protocol is defined in our DestinationView. If we had more information, we could either add methods or create a struct to hold all the data we need to pass.
We’ll create a property for the delegate, and when ever someone selects a color, we’ll tell the delegate the selected color. So who gets to be the delegate?
The calling ViewController of course! So we’ll make StartViewController the delegate. When we call prepareForSegue, we’ll set the delegate property to self. Then we make sure StartViewController conforms to the protocol by adding the setColor method. In the setColor method, we’ll set the background color of the subview to the color we’ve selected.
To return to the calling view, we’ll create events for when a user presses a button. In the event, we’ll call setColor and then we’ll pop off back to the root view controller. That’s the StartViewController in this case. Again, we’re not referencing StartViewController directly so we can call this from other places in our app if we need to.
And that’s how we pass data between iOS views using Swift 2.0. We pass information to the second view using the prepareForSegue method, and we pass information back to the calling view using the delegate pattern. It’s important to note delegate pattern examples usually use the calling ViewController as the delegate, but that’s not always desirable. You might have one class holding all the state for your application, and that would serve as the delegate. It depends on what you’re trying to accomplish. Any class that conforms to the protocol can be the delegate.
If you have any questions let me know in the comments. If you liked this video, like and share. Liking helps me attract more views. New videos come out each week, so make sure you subscribe. You don’t want to miss a video! Thanks for watching, and I’ll see you in the next tutorial!
<!-- DeegeU - Right Side -->
<ins class="adsbygoogle" style="display:inline-block;width:336px;height:280px" data-ad-client="ca-pub-5305511207032009" data-ad-slot="5596823779"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script></p>
Tools Used
- XCode
Media Credits
All media created and owned by DJ Spiess unless listed below.
- Music from YouTube free music.
- No copyright infringement intended.
Get the code
The source code for “How to pass data between iOS 9 views in Swift 2.0” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-ios-swift-segues.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Comments
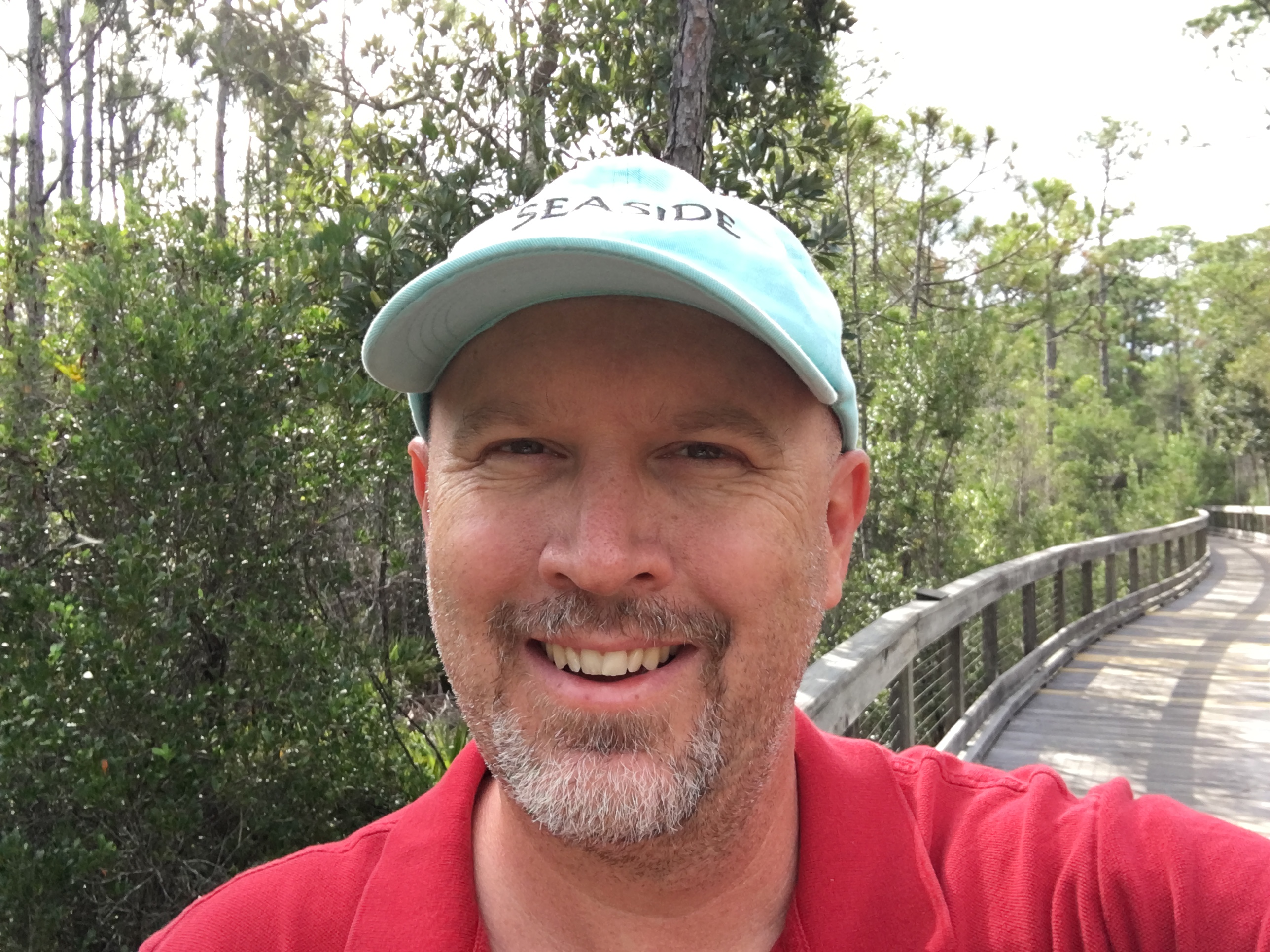
DJ Spiess
Your personal instructor
My name is DJ Spiess and I’m a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I’ve programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I’ve worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.