The Tools Used for Writing RESTful Web Services in Java
Tools for Writing RESTful Web Services in Java
This video introduces you to the tools for writing RESTful web services in Java. It was originally part of a larger unpublished video tutorial on creating JAXRS applications using Java.
Transcript
Hello! In this video we are going to look at the steps involved in creating and deploying a RESTful web service in Java. We will also note the tools used at each step, so you start to get an understanding of how each tool is used. This lecture will not be too detailed. It will be an overview to familiarize yourself with all the moving parts. We will examine each tool in depth in future videos.
Designing the interface
The first step in writing an application is deciding what your program will accomplish. You decide what services your API will provide, how it will return data, and determine what represents success or failure for each call. We will start with use cases to help us scope what information needs to be exchanged between the client and server.
Now this step does not have a tool per se, but I will provide you a template to fill out in future videos. This template will help you organize your API, because it will help you identify your resources, which are the nouns in your application, and identify which HTTP verbs apply to the resources. Basically this is the design step. In this step you’ll also want to think about how each resource should be returned. For example does it make sense to return the resource as XML and JSON, or is it returned as an image. You will also need to think about if your resource can be returned as a list or resources.
Write code in Eclipse
The second step in creating web services is writing the code. This is the fun part and the step where all the work happens.
You can write Java code in a text file using Notepad or some other simple text editor, but that’s painful. We will use an integrated development environment, or IDE, called Eclipse. There are other IDEs you can use, but in 90% of the development world you use either Netbeans or Eclipse. Eclipse has better ties into Arquillian and Wildfly, so we’ll pick Eclipse for this course.
Build code in Maven
After writing our code in Eclipse, the next step will be to compile it.
IDEs can be a blessing and a curse. You can set up your application to compile perfectly in the IDE on your computer, but then everything falls apart when you try to compile on another machine. You might be using slightly different versions of libraries, the wrong version of Java, or who knows.
Maven is a tool that helps you structure your project, knows how to compile your code, run your unit tests, assemble any artifacts, and maintains a database of dependencies your project might have. If you’re from a C/C++ world, Maven is like a make file on steroids. Maven will put all our code into a special application called a web application.
Maven also allows your development process to be repeatable. You should be able to hand off your code to another developer, and with a Maven script everything should compile. This is imperative in professional Java development, and all programs in this class will use Maven.
Deploy code to Wildfly
Once we have our compiled code, we need to find a way to run it. Earlier I mentioned web services are a collection of smaller programs. This means they aren’t executed like normal programs. Each smaller program can be the entry point to our application. They need to run inside a Java application container.
The Java application container is responsible for managing our web service. The Java application server provides many services for our RESTful application, most importantly it receives requests from the internet and hands them off to our web service application.
The web application container we will use is Wildfly. Wildfly used to be called JBoss, and really is JBoss 8. If you have experience with JBoss 7, you will find Wildfly very easy. If not, its still very easy.
Test code in Arquillian
Now that we have an application we can run, we need to test it. You should never write code without writing code to test it. We need to know the code works, and when we add new functionality, we want to make sure the old functionality doesn’t break. That’s why the fifth step is to run tests on your web services. The catch is to run these tests, we need to run the tests in the application container.
You might be familiar with testing Java code with JUnit. We will use something called Arquillian. Besides being named after an obscure Men in Black movie reference, Arquillian allows us to write JUnit tests that can run within a Java application container. We can make our changes, and see immediately that the code runs correctly and we didn’t break anything.
That is exactly what we need. Arquillian will take our application, deploy it to an application container, run the tests, and then remove the application from the application container. In the end we’ll get the test results.
Layers of application
Lets finish our discussion of tools by looking at the tools used inside our RESTful web service. For the most part, these will be other APIs we use within our code.
Client layer – HTTP and mobile apps
The client layer contains all the client applications that access our RESTful web service. Later in the course we will write code to call our service from web pages, Android applications, and iOS applications. First we need to create an API to use.
For web pages, we access our web services using Javascript. In android applications we’ll write Java code to consume our web service, and in iOS we’ll write code in ObjectiveC using XCode.
Presentation layer – JAX-RS and Resteasy
We handle requests to the web service in the presentation layer. This is where we will convert resources to the formats we support. This can be XML, JSON, an image, or anything else we think up. You really can return anything from the web service. Well almost anything.
We will also map the requests to specific points in our application called endpoints. Ironically these are the points where smaller web service applications start. A endpoint can be the individual call to perform some action on a resource. We will map these endpoints using the Java API for Restful services, or JAX-RS.
JAX-RS is just a specification. For now you can think of it as having a similar relationship a interface has to a class. It defines how the API should work and what functions it should provide, but it is not an implementation we can use. We need to use an API that implements the JAX-RS specification. In our case, we will use Resteasy.
Resteasy was picked because it comes packaged in Wildfly and implements JAX-RS 2.0. And like the name implies, it’s pretty easy to use.
Domain layer – EJB
The resources returned in the RESTful application need to be mapped to data objects. We also need domain specific logic, or business logic. The domain layer contains the real world rules for creating, updating, retrieving, and destroying data. We program this layer using EJBs and plain Java code. The domain objects represent our data as how the client views it.
Data layer – JPA and Hibernate
Almost every useful web service application needs to read data and store data. To make our lives easier we’ll use the Java Persistence API. This is another specification, and the implementation we will use is Hibernate. These were chosen again because they come standard in Wildfly. The data objects in our API represent our data as how the database represents it.
Data store – MySQL
Finally we need a database. We will use MySQL because it’s free, easy to set up, and very popular. Since we’re using JPA to abstract most of the database from our view, you could eventually swap out MySQL with any other database that Hibernate supports.
OK, that was quite the whirlwind tour of the different tools we will use to create our RESTful API. In the next video let’s dive in and start using these tools. The first tool we will cover in depth is Maven.
Tools Used
- Java
- Maven
Media Credits
All media created and owned by DJ Spiess unless listed below.
- No infringement intended
Get the code
The complete video lesson list for this free online programming course can be found on the course syllabus page.
The source code for this video can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/maven.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Don’t miss another video!
New videos come out every week. Make sure you subscribe!
Comments
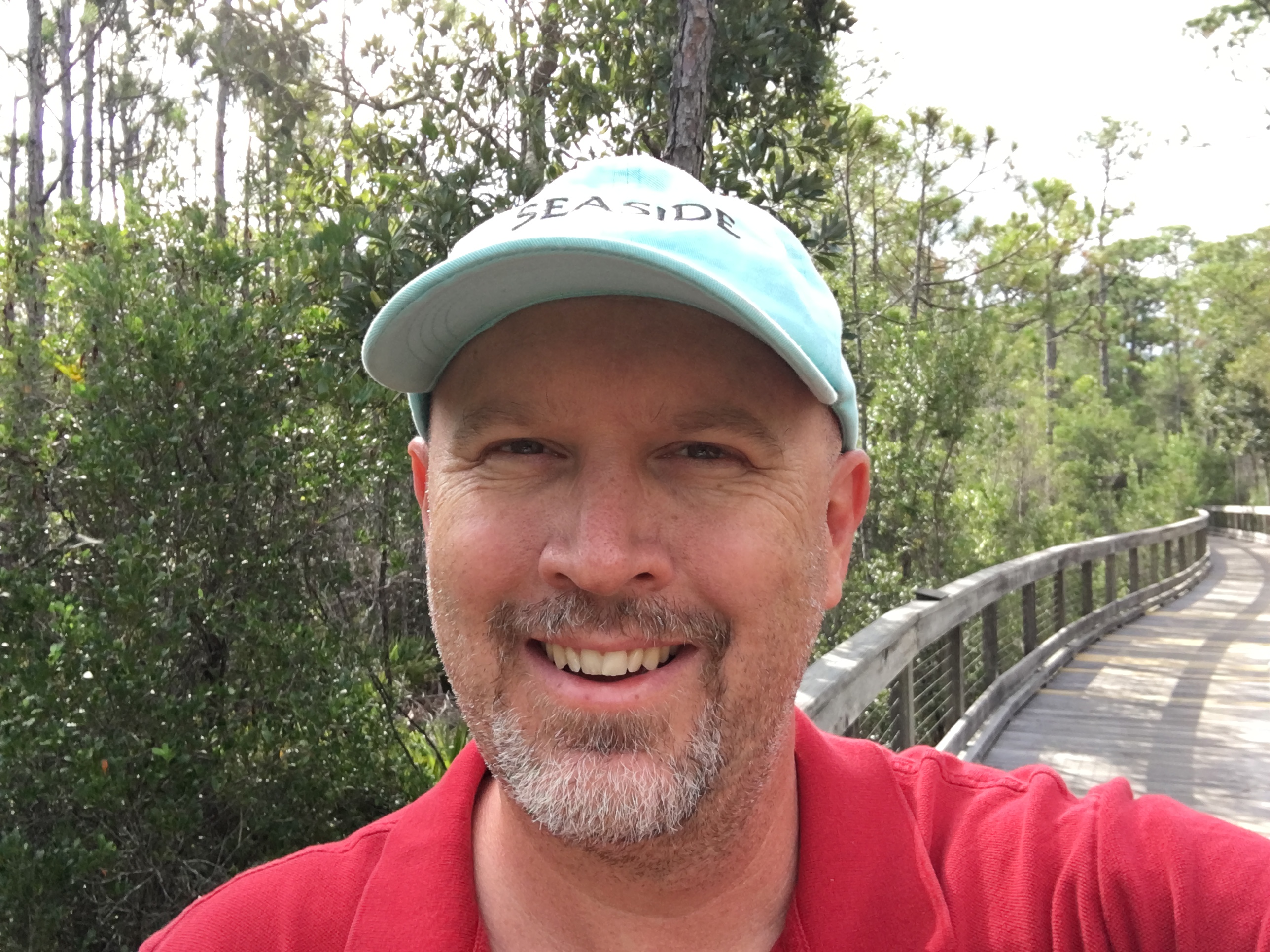
DJ Spiess
Your personal instructor
My name is DJ Spiess and I’m a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I’ve programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I’ve worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.