Watch this Java String Tutorial! – J050
DeegeU Java Course
The “Watch this Java String Tutorial!” video is part of a larger free online class called “Free Java Course Online”. You can find more information about this class on “Free Java Course Online” syllabus.
Transcript – Watch this Java String Tutorial!
In this video we’re going to start looking at strings. Strings represent the text we use in applications. We’ll start with the basics in this tutorial, and over the next few videos we’ll cover more complex string topics.
What is a Java String?
Strings are a collection of characters strung together into a single datatype. That’s why they are called strings. We can think of them as words and sentences. They’re important because humans don’t think directly in numbers. We need characters and words for our programs.
In Java, strings are represented using the String class.
Creating strings
Strings can be declared similar to numbers using literals. That looks like this. We simply declare our string variable, and give it a literal value.
We can also declare a string using a constructor. We’ll rarely ever see this method of creating strings. The reason is the literal is a class instance. So this code is really creating a string instance using the literal and passing the instance to the constructor to create another string. It’s double work creating two strings. Doing this in a loop a billion times could create big problems for us, since we’d be creating twice as many string instances as necessary. That would also create headaches for instance garbage collection.
The String class let’s us use and manipulate strings. For example, we can declare a string like this. Then we can call a method on the String class. In this case, we’re getting the length of the string.
String name = "Dan"; int nameLength = name.length();
String literals are also string instances. So we can do some interesting things syntactically, like call a method on a literal.
int nameLength = “Dan”.length();
Either way works, and it really depends on the specific case as to which form makes more sense. Usually we wouldn’t call length on a string literal, since we should know the length at that time.
A better example is testing a string for equality. We might want to check is a string is equal to a known literal like this.
String name = "Dan"; if (name.equals("Dan")) { // Do something }
However, if the string is null, we’ll get a null pointer exception. A better check is to use the method on a literal like this.
String name = "Dan"; if (“Dan”.equals(name)) { // Do something }
The literal will never be null, and if the value is null the equals method will just return false.

Java String pool and testing equality
Another thing we need to know about strings is Java tries to help us conserve memory by doing something called string interning. What this means is Java recognizes when we do something like this. We create a string a and a string b, both with the same literal value.
If we test for equality using the equality operator, the test is true. But we need to be careful here. This doesn’t mean the two strings have the same value. We use the equals method to test that. The equality operator means a and b reference the same string instance. The reason is Java creates a special pool of strings, and reuses the instances when it can. This is called string interning.
If we deliberately create a string using the constructor, we’ll get different results. The reason is the string created using the constructor does not use the interned instance. It creates another instance. So in this case, a and b point to different instances.
Java Strings are immutable
Strings are also immutable. This means once we create an instance, that’s the value. We can’t change it. Changing the value of a string variable like this, creates a new instance.
There are several reasons strings are immutable. One reason we just saw is interning in the string pool. If we could change the value, we’d be changing the instance for every place the string was used, which would defeat our ability to cache strings.
Another reason is security. Imagine we have a method that takes a string representing a query to a database. If we could change the string, we could wreak havoc in our database. Or secretly connect to another database. It would be bad.
There are other reasons to make strings immutable such as thread safety, and use in other classes like the Sets and Hashtables. We’ll cover these more later.
Conclusion
So that’s the quick look at Java strings. We saw how to create Java strings, how to test if two strings are equal, and we noted that strings are immutable. If you have any questions, add them to the comments below. We’re going to look more at strings in the next few videos, since they are very important for most Java applications.
If you enjoyed the video, please share and like. And with that, I’ll see you in the next tutorial!
<!-- DeegeU - Right Side -->
<ins class="adsbygoogle" style="display:inline-block;width:336px;height:280px" data-ad-client="ca-pub-5305511207032009" data-ad-slot="5596823779"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script></p>
Tools Used
- Java
- NetBeans
Media Credits
All media created and owned by DJ Spiess unless listed below.
- No infringement intended
Whatdafunk by Audionautix is licensed under a Creative Commons Attribution license (https://creativecommons.org/licenses/by/4.0/)
Artist: http://audionautix.com/
Get the code
The source code for “Watch this Java String Tutorial!” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-java-intro.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Comments
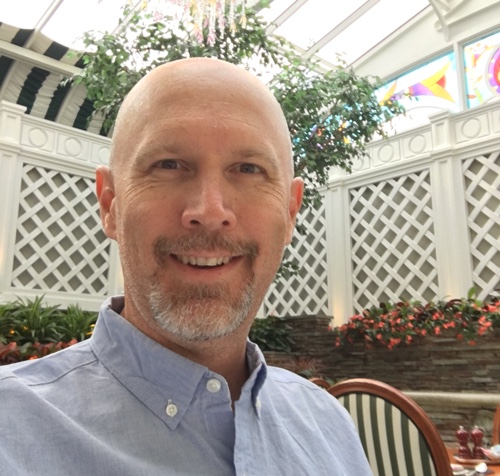
DJ Spiess
Your personal instructor
My name is DJ Spiess and I'm a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I've programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I've worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.