The Java Operators Tutorial Video (part 2) – J015
DeegeU Java Course
This video “The Java Operators Tutorial Video (part 2)” is part of a larger free online class called “Free Java Course Online”. You can find more information about this class on “Free Java Course Online” syllabus.
Try at home
- Try testing if different expressions are equal and not equal
- Try testing the difference between strict inequality and just inequality
- Try shifting numbers to the left and right
- Try using the XOR operator to XOR a value, and then XOR again to get the original value
- Try creating errors in your program and compiling it
Transcript – The Java Operators Tutorial Video (part 2)
In the last lesson, we focused mostly on math operators. Numbers are important, but we need to construct larger and more complex logic operations, like hey is my shape a circle and is it red? We also need to perform bit manipulation. And that is what we are going to cover in this lesson!
The goal for this lesson is to finish up our understanding of Java operators. We need to cover the equality operators, logical operators, and bit operators. Then we’ll take a final look at operator precedence.
Java equality and relational operators
Equality and relational operators check to see how two values are related. These operators all return a boolean value. Remember a boolean value is either true or false. So each of these evaluates to either true or false.
To determine if two values are equal, we use two equals symbols for equality. This is different than the assignment operator. That uses only one equal sign, and it’s a common mistake to use one equal sign when you mean two. Luckily Java will complain about it when you try to compile. I’ll show you that when we get to the code. In this example, isEqual would return false.
We can also test if two values are not equal. That uses the exclamation point with the equals sign. We read that as A is not equal to B. If they are not equal, the test returns true. This is the opposite of the equality operator. 1 not equal to two would return true.
We use the greater than and less than symbols to test strict inequalities. A strict inequality says, is A strictly greater than B. The answer is always true or false. If A = B, then the strict inequality is false. For this example, 1 greaterThan 2 will be false while 1 lessThan 2 will be true. If both values were 1, 1 greater than 1 is false.
The not so strict version is, is A greater than or equal to B or is A less than or equal to B. In this case, we’re allowing equals to return true as well. If A equals B, then the operator returns true. We use an equals symbol with the greater than or less than symbol for these operators.
Lets look at assigning booleans to equality and relational operators.
So if we set A equals to 3 and B equals to four, lets see what happens for each operator.
Equals is false.
Not equals is true.
Greater than is false.
Greater than or equal is false.
Less than is true, and less than or equal is true.
Now lets set A and B equals to four and run it again.
In this case
Equals is true.
Not equals is false.
Greater than is false.
Greater than or equal is true. This is because the two values are equal, so that counts as true in the test.
Less than is false, and less than or equal is true.
Now I said earlier that it was a common mistake to use a single equals. Lets see what happens if I delete one of the equals signs in the first test. It won’t even compile. It complains with “int cannot be converted to a boolean. That’s because it actually is trying
to do the assignment chaining we saw in the last lesson. It’s setting A equal to B, then trying to assign it to the boolean value. The int is not a boolean, so we get an error.
Java conditional operators
Next up are conditional operators. Conditional operators perform logical operations on boolean values. You may know these as AND and OR.
And is the first one we’ll look at. It reads like an English statement. A and B. If A is true and B is true, A and B is true. If either are false, then A and B is false. Both A and B must be true for the result to be true.
Or also reads like an English statement. A or B. If A is true or if B is true, then A or B is true. If A and B are both false, then A or B is false. Only one, either A or B, needs to be true for the result to be true.
Finally there is one unary operator we didn’t cover in the last lesson. It’s called “not”. So if B is true, not B is false. If B is false, not B is true. Basically the not just flips the sign of the boolean value. We use an exclamation point in front of a boolean value or expression for nots. When ever you see the exclamation point, you can substitute the word “not”.
Lets look at the boolean operators.
Assume A is true and B is true. Here’s what AND, OR and NOT look like.
Now lets make B false and see what they look like.
Now the power with boolean expressions is we can chain several of these together. We can also use equality and relational expressions. So assume A is true and B is 4 > 3. Lets play with that a bit.
Note these can get long.
The equality operators will be evaluated first, then &&, then ||. You really don’t want to string them together like this how ever. It’s not intuitive to know which operators have precedence. You want to be explicit about the order statements are evaluated. To do that, use parentheses. We’ll look at this closer in a minute.
Java bit operators
Finally we have the bit operators. These operators are for changing the individual bits of a integer number. Remember integers include bytes, shorts, int and longs. The first group of bit operators we’ll look at are the bitwise operators.
The bitwise operators are for AND, OR and exclusive or (XOR). These take two integer types, and return an integer or a long. That’s important. If you use these operations on two bytes, or two shorts, the result will be an int. The result is always an int or a long, unless you cast it back down.
The bitwise operators are similar to the boolean operators we saw before. In fact, you can mentally think about these operations as 1 is true, and 0 is false as you work through each bit in the number. We call a bit that has a 1 as set, and a 0 as clear. The bitwise operators use a single ampersand for and, a single bar for or, and a tilde for a new operation called exclusive or.
Lets assume we have two bits we want to AND. We’re saying if the first bit is 1 and the second bit is 1, then the result from an AND is 1. If we had a 1 and a 0, the result is 0. ANDs want both to be a 1, otherwise it’s a zero.
We can extend this for every bit in an byte. If we AND each of the 8 bits in a and b, we’ll get the following result. We get set bits only in the positions where the a and b bits were also set.
ORs are different. For an OR we are saying if the first bit is a 1 or the second bit is a one, then the result of the OR is a 1. If they are both a 1, it’s still a 1. For ORs, the answer is a 1, unless both initial bits are a 0. Again this can be extended to a whole integer.
Here if we OR each of the 8 bits in a and b, we’ll get the following result. We get set bits only in positions where there is a set bit in a or b.
Exclusive or is spelled XOR and uses the carrot symbol. This behaves like an or, except if both are a 1. In this case if both are a 1, the result is 0. It’s the Highlander bit operator. There can be only one one. It’s exclusive. That’s how I remember it.
Here if we XOR each of the 8 bits in a and b, we’ll get the following result. We get set bits only in the positions where a bit was set in only a or b, but not both.
One unary operation we skipped in the last lesson is the bitwise unary not. We skipped it, because I wanted to keep all the bit manipulating operations together. The unary not flips all the bits in your integer. So if the bit is 1 it becomes 0, and vice versa.
Here if we NOT each of the 8 bits in a, all the bits get flipped. Set bits become cleared bits, and cleared bits become set bits.
Lets look at some code for this, and hopefully it will become clear.
We’ll work with integers. We’ll set our first number to all 1s. The second number will be half ones, and half zeros. If we AND the numbers, we’ll get back a number with half ones, half zeros. Basically we got the same number back. If we OR the numbers, we’ll get all the bits set. This is because all the bits are set in the first number.
Things are a bit more interesting for XOR. When we run this, we get the reverse of the second number. Look at what happens if we set the first number to 42, and the second number to 24. We XOR the numbers and get 50. We then XOR 50 with the second number again, we get back to 42. You can do this with any two numbers.
And for completeness, here’s how we flip the bits using the not.
Java bit shift operators
These operators are used for bit shifts. What does that mean? It means we move all the bits to the left or right, depending on the direction of the arrows.
These are the operators used for bit shifts. The direction the symbols point determine the direction of the shift.
If we are shifting right, the most significant digit in the number determines what is shifted in. So if the highest digit is 1, we shift 1 into the number. If its a zero, we shift zero. The reason for this, is we are keeping the number either positive or negative. As you remember, the highest bit determines the sign of the number. We saw that in the how computers store negative numbers lesson.
Sometimes we always want to shift a zero into the number, so for that we use the unsigned shift right. That has three greater than symbols. A zero is always shifted in. Again, let’s play with these in code.
We’ll take a number, and shift it to the left. The cool thing to note here is, the result is just the previous number times two. So when you shift left, each shift is a power of two If we shifted by 2, the number is multiplied by four.
Shifting right is just going in the opposite direction. Let’s do one negative number, and one positive number. As you can see, the shifting keeps the sign.
If we do it again with the unsigned shift, you see the sign changes for the negative number. That’s because we shifted a zero in, making it positive.
If you want to see more bit operations, check out 4 ways to count bits in a byte. Manipulating bits is a common interview question, although the types of programs you work on will determine how often you work with bits.
Java operator precedence
The last thing we wanted to discuss was operator precedence. We’ve covered all the Java operators individually. You can chain many of these into larger more complex operations. The catch is Java will perform operations in a specific order. It’s not always left to right. Here’s the list of operator precedence.
The way to read this chart is, Java will start from the top, and work it’s way down. If operators are on the same line, it will work from left to right. Again, you want to use parentheses to make it explicit.
It’s not a good practice to chain long operations without parentheses. If your expression is really long, you might want to break it up into smaller statements like this. That way there’s no ambiguity in what you’re trying to calculate. Parentheses always goes first.
And that wraps it up for the different operators. We now have the power to control our numbers down to the bit level. We can form complex boolean expressions using the logical operators, and we know how to test for equality. We can now start using these operators to build bigger programs. Programs that actually do something.
You’ll want to play with these in your own Java programs. It’s easier to understand when you see it happen in code. If you have any questions, leave them in the comments below or on DeegeU.com.
See you in the next lesson!
Tools Used
- Java
- NetBeans
Media Credits
All media created and owned by DJ Spiess unless listed below.
- No infringement intended
Get the code
The source code for “The Java Operators Tutorial Video (part 2)” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-java-intro.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Don’t miss another video!
New videos come out every week. Make sure you subscribe!
Comments
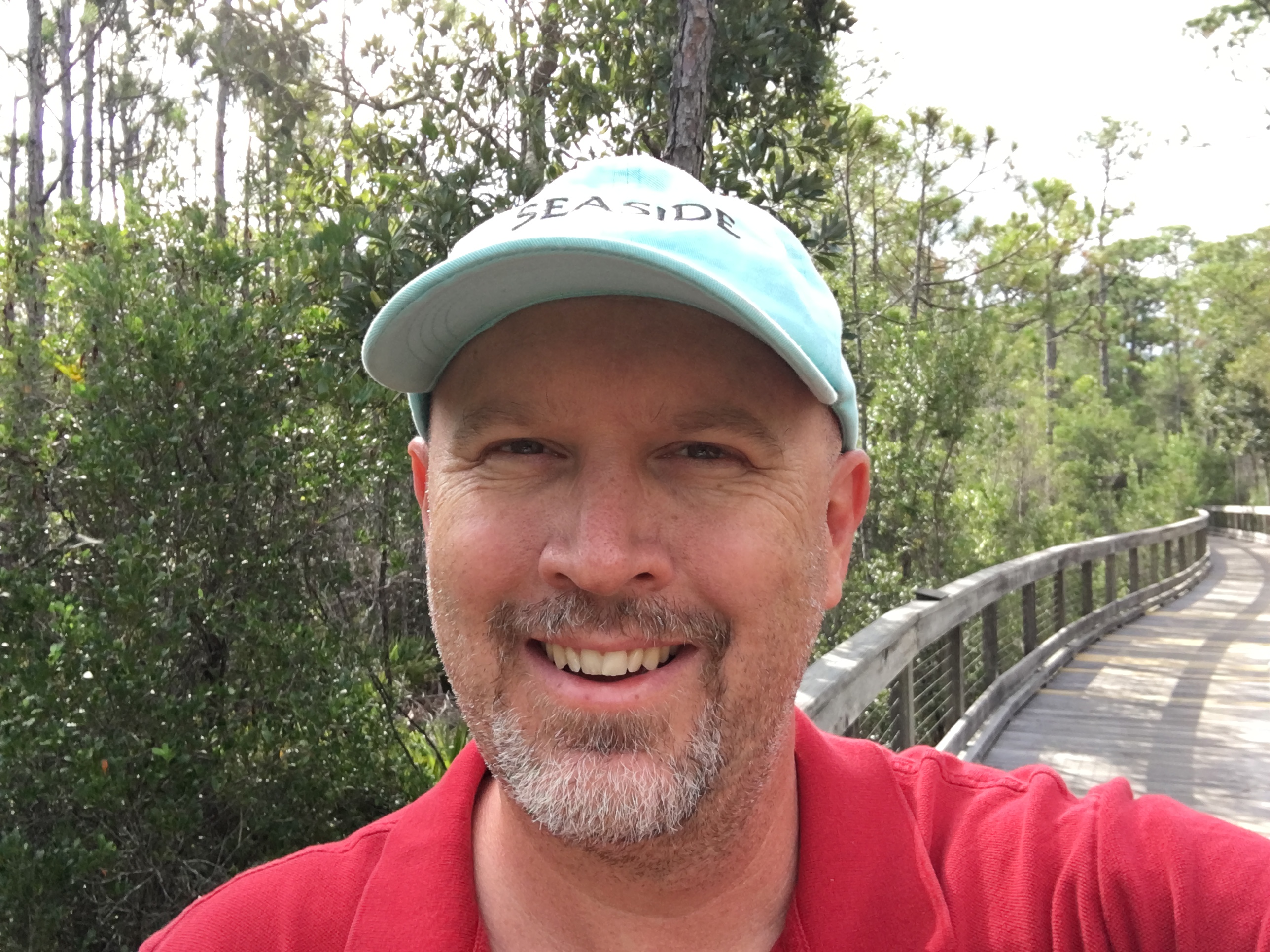
DJ Spiess
Your personal instructor
My name is DJ Spiess and I’m a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I’ve programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I’ve worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.