The Java If Else Tutorial Video – J017
DeegeU Java Course
This video “The Java if else tutorial” is part of a larger free online class called “Free Java Course Online”. You can find more information about this class on “Free Java Course Online” syllabus.
Try at home
- Write an if-then statement that checks if a temperature is greater than 25 C.
- Write a few larger if-then-else statements
- Write your own game using the if-then (you’ll need the println and Scanner from lesson 2)
Transcript – The Java If Else Tutorial
Growing up I was pretty nerdy. I loved computers, games and comic books. When I first started out programming, I wanted to
make games. I made my first game ever when I was 10 based on my favorite comic book, Green Lantern. The game was really simple. It was a text based game where you were presented with a choice. If you made the correct choice for our hero, you’d continue in the game. If not, Green lantern died. If you got past all three choices, you won. The full game used simple print statements, and the if-then statement. This is what I’m going to show you next.
The goals for this lesson are to understand the if-then-else control statement. As we have in past lessons, we’ll look at ways the code go wrong. This way you know how to identify the errors in your code when they happen. We’ll touch upon short circuit evaluation, and then when we’re done looking at the normal if-thens, we’ll quickly look at the very succinct ternary version. Its great for setting single values without writing whole if-then. So let’s dive in!
An if-then is a Java control statement that behaves exactly how it sounds in English. If this statement is true, then do this. The if-then has a keyword and a condition. The keyword “if” starts the control statement. It is immediately followed by the condition to test. The condition is always enclosed in parentheses. It’s an error to try to create the if-then without parentheses. If your condition is true, the java block is executed. False, and the java block is skipped. We can test, if ring is charged, shoot bad guy. If the ring isn’t charged, then the code won’t run.
The condition can be a boolean expression or a boolean variable. The only requirement is anything inside the parenthesis must evaluate to either true or false. If you’re coming from a C/C++ background, it is important to note that the condition does not evaluate to 0 or 1. It must be a true Java boolean type.
Since it can be a boolean expression, you can also do something like this. If isVisible is true and power is greater than 5, run this block. Both conditions must be true. It is a good idea to use parenthesis inside the condition expression to make it clearer.
Larger conditions should be compressed for readability. You can create a boolean value from a complicated expression, and then just test the value. In this case we are creating a variable called myCondition, and setting it to the result of the expression. Lets play a bit with if-then statements so we can get these ideas clear.
Sometimes we want to say if something is true do this, other wise do something else. We have that ability in Java. We can add another keyword called else. In this example we are saying if a is greater than 5, do this java block. Otherwise do this other java block.
You can also chain these into longer if-then statements. For example, this block tests if a is greater than 5 and then tests if a is less than or equal to 5 if a is not greater than 5. This really is the same thing as the else statement from before, we’re just making the second test explicit. If you use the keyword else without a condition, it will run the following Java block if all other ifs are false. You can add as many else-if as you need as well. Just don’t go too crazy, or it gets hard to read. Lets look more at the else statements.
So what happens when not everything is accounted for? For example, in this case there is a block for a greater than 5, and a block for a less than 5 (PR). What happens when a equals 5. Well this just means that all the code blocks are skipped. Nothing is run. This illustrates the importance of checking all your boundary conditions when working with numbers and inequalities. If you are ignoring the equals case, this would be a really good place to add a comment explaining why. Otherwise you’ll forget, or someone else looking at your code will be confused.
Another common source of errors is forgetting to use braces. As we saw earlier in the blocks lesson, a statement can be a block. Still you want to use braces. Here’s a good example where you might not get the behavior you’re expecting. In this example, removing the braces means the second line will always be printed. The indentation is ignored. Going back to the IDE, we can see this problem in action.
Sometimes you’ll have a test that takes much longer to evaluate, compared to the other tests. For example you might test if 5 is somewhere in a list of a million numbers, and test if b is larger than 4. The test for b is much easier and quicker than searching through a list of a million numbers. If both need to be true, always test the easier test first.
In this case it would be better to switch the boolean tests. This works because of short circuit boolean evaluation. This means it will stop evaluating the boolean expression, if it knows it will evaluate to false regardless of the rest of the expression. Since both need to be true, there’s no point in searching a million numbers if b is not greater than 4. So if b is 2, Java won’t even try to search.
When you just need to set a value based on a boolean expression, there is a simpler code than the full if-then. This is called the ternary operator. It’s an operator we didn’t cover in operators, because this operator is a control operator and we hadn’t covered what that meant yet.
You can write it in a one line statement. You simply set your variable to an expression. The first part is the expression to test. Next we use the question mark to note that this is an if-then test. Finally we put the two possible values separated by a colon. If the condition is true, the variable is set to the first value. Otherwise it is set to the second value. There is no possible way to handle else in this expression. Let’s go play with this in the editor.
There are ways you can really abuse the if-then statement and create some obnoxious code. For example, you can create this if-then code to set a boolean value. This is bad form. The reason is this is 6 lines of code, all to set a boolean value. Can you guess the better form? I’ll give you a hint, it’s not the ternary operator
This is what you should do for booleans. In this case, the boolean expression you’re evaluating is a boolean value. Instead of using an if then to do logic, just set it to the value and you’re done. The best code ever is the code you don’t write.
So in this lesson we learned about the if-then-statement and how we can use it to control what code is run in our application. We learned how Java will short circuit your conditions, and how this can speed up your program. We also learned about the ternary operator.
And with that I’ll see you in the next lesson!
Tools Used
- Java
- NetBeans
Media Credits
All media created and owned by DJ Spiess unless listed below.
- Green Lantern owned by DC Comics (http://www.dccomics.com/characters/green-lantern)
Get the code
The source code for “Are you ready to tackle the fizzbuzz test in Java?” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-java-intro.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Don’t miss another video!
New videos come out every week. Make sure you subscribe!
Comments
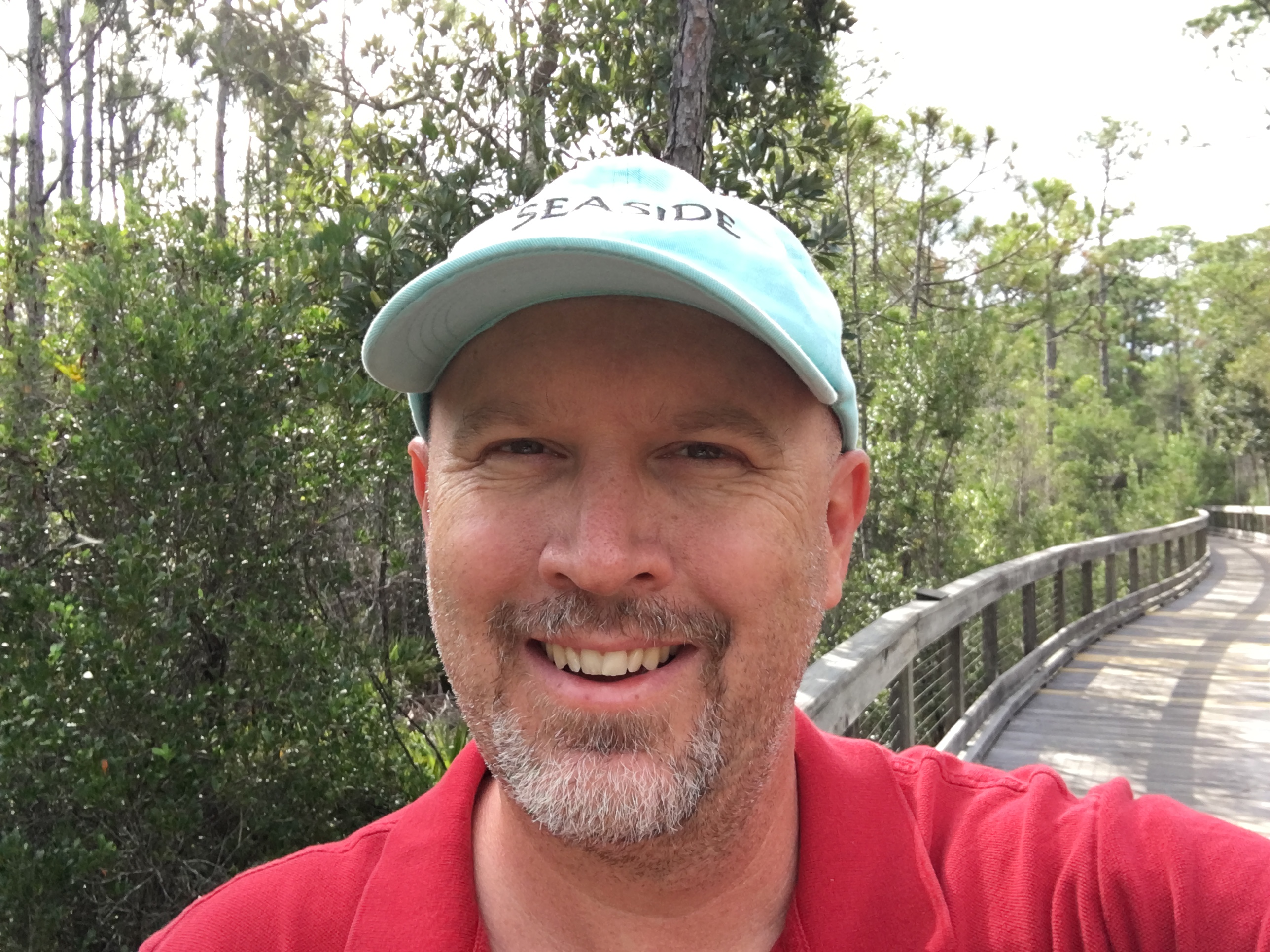
DJ Spiess
Your personal instructor
My name is DJ Spiess and I’m a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I’ve programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I’ve worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.