How to create Java arrays tutorial – J013
DeegeU Java Course
This video “How to create Java arrays tutorial” is part of a larger free online class called “Free Java Course Online”. You can find more information about this class on “Free Java Course Online” syllabus.
Try at home
- Type in a program creating an array using new
- Type in a program creating an array with literals
- Type in a program creating a multi-dimensional array
- Type in a program that copies an array
- Type in a program that sorts an array using java.util.Arrays (bonus)
- Type in a program that searches for a number in your array using java.util.Arrays(bonus)
Transcript – How to create Java arrays tutorial
Imagine someone has asked you to create an application that reports the average, high, medium and low temperature for your city. You can create three variables for each month. This would give you 36 variables to keep track of. That’s three temperatures, times twelve months. Determining the average low temperature for April would mean you’d need to have special code to match the selected month with the three variables for that month. That sounds like a lot of work.
Luckily there’s a much better way that I want to show you. Java has a data structure called arrays.
Here your goals for this lesson are you want to understand what an array is, and what it represents and what it looks like. You need to know how to create arrays in your Java code. And once you’ve created one, you’ll want to know how to get the individual elements in an array. Its important to know how to copy arrays so I’m going to teach you how to copy arrays and there’s a whole list of tasks you can do with an array, and job has provided many of them for you. You want to know how to use these tools in java.util.Arrays.
In memory an array occupies a region of memory. You can think of each memory location as a mailbox, each with its own address. In a previous lesson you learned how to store a single value in a variable. The variable is held in a single memory location. For our weather example, we don’t want to create 36 distinct variables. When we need to hold a larger ordered group of variables, all the same type, we use an array. You can create an array any type including an array of arrays. We’ll look at that later, but first lets look at a simple array.
Here’s an array of eight elements. Each element in an array is next to the previous element, ordered from the least to greatest address. One of the key characteristics of an array is that it’s a fixed-length. If you specify an array to hold 8 values, like this one, it will not grow when we try to add a ninth element. For an array with eight elements, the index for each element is from 0 to 7. The first element always starts at zero. That’s why you always see programmer jokes like this.
Get it? First element is 0.
Isn’t that hilarious? Ha Ha. Alright just to make things clear, if we populate our array with random variables like this, the sixth element which has an index of 5 has the value of 67. Let’s look at the Java statement required to declare an array. It starts with the Java type. This can be any Java type. In this case long. The type is followed by two square braces.
The braces tell Java that you want an array of this type. Variable name is like any other variable name. You can call it whatever you want. Make a good name! Since we’re starting with the temperature app, let’s call it high temperatures. Then you have a choice of how you create your array.
Place holders or literals. The first way is to use a “new” keyword. That looks like this. We specify the array type again, but this time we include the number of elements. This is the true number. If you want 10, put 10. This statement will create a Java array with 12 longs for elements. The statement is saying declare an array of longs, call it highTemperatures, then create an array of 12 longs, and assign it to high temperatures.
It’s like when you create a normal variable and assign it to a value, the type is the array of long. The value is a particular array with 12 longs in it. The index to each element will run from 0 to 11. You can also break the declaration in creation up. This is can be useful if you want to create your array with some time after declaring the array. If you know in advance what values for each element will be, you can also create the array from a list of literals. That looks like this.
In this case you don’t need to tell Java how many elements are in your array. Java can figure it out. For this it would create an array of size 2. Let’s go to the Java editor and write our three temperature arrays. We’ll create one for each temperature range: high, average, and low. Let’s start in usual starting place.
So what we want to do is create three arrays, each has 12 elements. One for each month. The first to store the monthly low temperatures. Second stores the monthly average temperatures. And the final array stores the monthly high temperatures. Now let’s print out the high, medium, and low temperatures for May.
May is the fifth month, but remember arrays are zero-based. That means they start at 0. So the index value would be 4 for May. We run it, and we get the values for May. You need to make sure you have the braces in place. Here’s what it looks like if you forget a brace in your array. If you forget the first brace, you get the error “int cannot be converted to an int array”. If you forget the closing brace, it says you forgot your brace.
What happens if you try to access a non-existent value. For example, assume you forgot that’ it’s 0 based and try to access December using 12. Well Java has no problem at compile-time. 12 is valid index even though it’s not a valid index for this array. So no compiler errors. We run it and we get a runtime error. The runtime error says java.lang.ArrayIndexOfBoundsException. If you look close, it even shows you the value tried to use.
In this case 12. Next thing I want to show you is how to copy an array. Now you might think that we can just copy the array by setting the new array to the existing array using equals. And that kinda makes sense, but you’d be wrong.
Look what happens if we copy an array, set the fifth value to 42. Now lets print out the first array. This is a special call to print arrays. Just know that it works when we use it. Methods are coming in later lessons. So the first array change too. That isn’t what we wanted.
The reason is we copied a reference. That’s a topic that we’re gonna cover later. In our mailbox analogy we said a mailbox holds one type and one type only. The type that we’re holding is an array reference, not the values in the array. Just the address to our mailbox. So we defined an array reference, then created an array and said our first reference points to that. Then we defined a second reference and pointed at the same array. So how do we copy the arrays? We could copy each element over into a new array but that would get annoying real quick. If we had millions in our array, even if we wanted to type each copy, that would take forever. There’s gotta be a better way. There’s a better way.
We’re going to use more stuff we don’t completely understand until we get to Java classes. Java has a special API for array manipulation called Arrays. It has a call that moves arrays around in blocks, which is much more efficient. When we want to copy an array, we just use this copy call. So let’s try that. Let’s go back to our problem.
We have two arrays, and we tried making a copy. We’ll change the assignment to call Arrays.copyOf instead. We run it again and look! Changing an element in the second array does not affect the first. Now I said earlier you can have a multi-dimensional array. That’s really just a matrix of values ordered like this. To declare you just add another set of square braces. That looks like this.
Let’s go create a multi-dimensional array in code. We create a square array using the following syntax. There’s really no magic to this. We can create arrays of any dimension. We just keep adding more braces to the creation. We can create multi-dimensional literal arrays too.
Let’s alter our temperatures array to be one multi-dimensional array. We’ll modify our array literal like this. What we’re doing is putting arrays as the elements in our array and then those arrays have ints. So now we can use one array to store our temperatures. 0 will be the index for low temperatures, 1 will be the average temperatures, and 2 will be high temperatures. There’s many other things you can do to manipulate arrays.
I encourage you to go experiment with the different calls and see how they affect the array. This will also give you more experience writing Java programs. If you need any ideas of what to try, check the lesson page on DeegeU.com. Let me know if you run into any issues down on the comments, and I’ll see you next lesson.
Hey! Thanks for watching the video. There is a quick quiz for this on DeegeU.com if you’d like to gauge how much you learned. If you like the videos you are seeing, please let me know by liking the video and hitting the subscribe button to the DeegeU channel on YouTube. I’d really appreciate that. If you have concerns or questions, please leave them in the comments below or on DeegeU.com. There’s a poll on the front page of DeegeU.com so you can also let me know what topic gets covered next. Thanks for watching see you in the next video!
Tools Used
- Java
- NetBeans
Media Credits
All media created and owned by DJ Spiess unless listed below.
- #0 Programmer mug from http://www.zazzle.com/worlds_0_programmer_mug_basic_white_mug-16805801958368225
Get the code
The source code for “How to create Java arrays video tutorial” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-java-intro.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Don’t miss another video!
New videos come out every week. Make sure you subscribe!
Comments
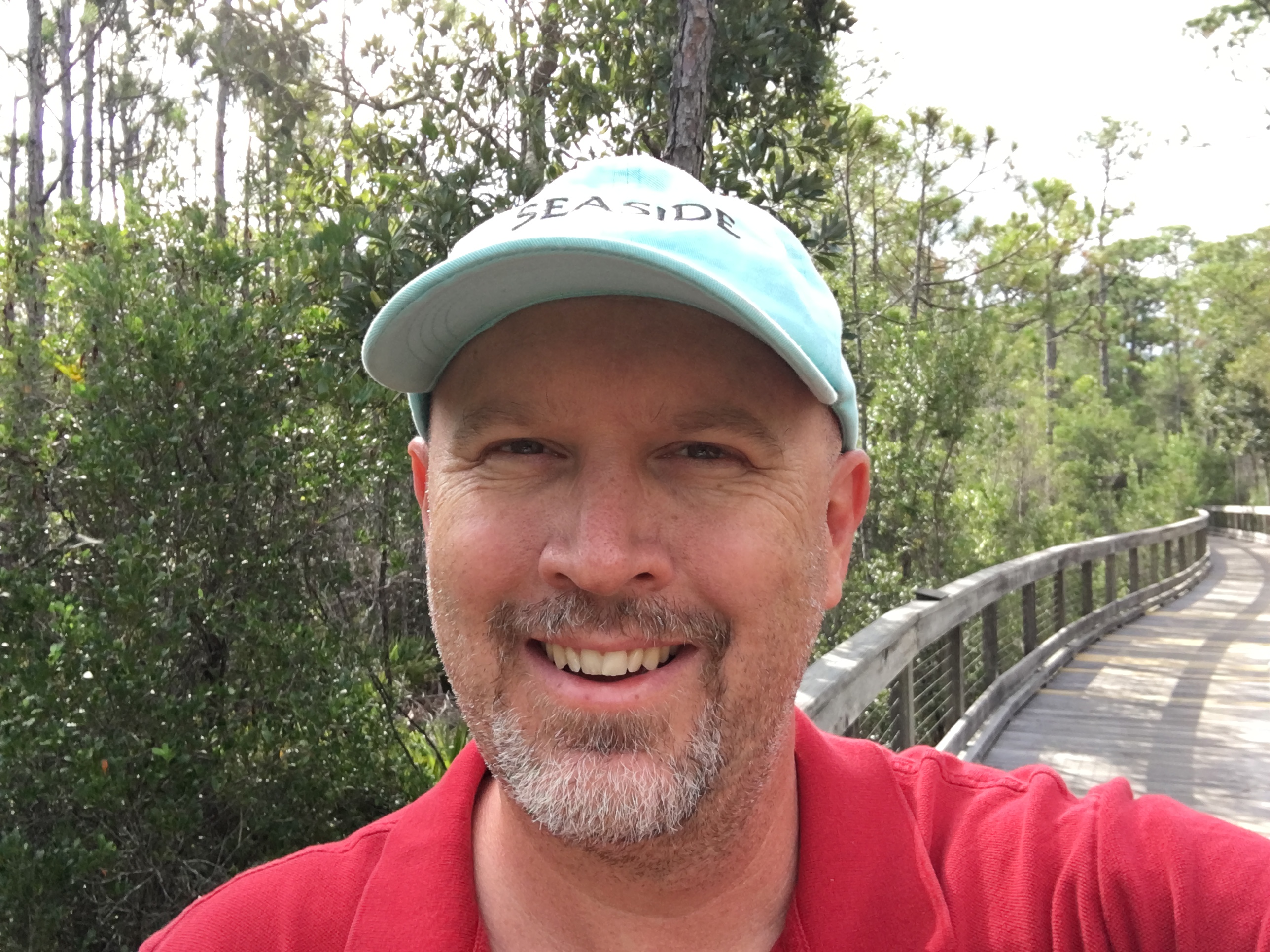
DJ Spiess
Your personal instructor
My name is DJ Spiess and I’m a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I’ve programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I’ve worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.