Java Bit Manipulation using the Java Integer Class – J049
DeegeU Java Course
The “Java Bit Manipulation Using the Java Integer Class” video is part of a larger free online class called “Free Java Course Online”. You can find more information about this class on “Free Java Course Online” syllabus.
Transcript – Java Bit Manipulation Using the Java Integer Class
In this tutorial we’re going to look at Java bit manipulation using the Java Integer class. Bit manipulation is used in error correction, compression, encryption and optimization algorithms. It’s one of those things that’s really important, or you won’t see it at all. But it’s really important.
There are many operators we use to manipulate bits, but as we saw in previous Integer class tutorials they don’t work for instances. The operators are meant for primitives.
To fix this, Java provides several methods for manipulating bits in the Integer class. Let’s take a quick look at all the methods available.
Counting bits in the Java Integer Class
The first one is bitCount(). At first we might think this tells us the number of bits in an Integer, but it’s really a count of the set bits. That means all the ones. So for this number (10001), the bitCount is 2. That’s because the first and last bit are set, and the rest are cleared.
When we’re counting bits, it can be useful to know the highest bit set. In one algorithm, it reduces the number of tests. The highest bit set is the furthest set bit to the left. So back to our number (10001), the highest value is 16. This is because the sixteenth place has the set bit. The method is called highestOneBit().
We can get the lowest bit set as well. This method is called lowestOneBit(). Using the same number, we’d get the answer 1. That’s because the left most bit set is the ones place.
To get the number of leading zeros, we’d call numberOfLeadingZeros. This will tell us how many zeros in the binary number our Integer starts with. So 294967295 looks like this in binary (10001100101001101011111111111). The first bit is a sign bit, so it doesn’t count. The next three are zeros, so this method returns the number 3.
We can also get the number of trailing zeros using the method numberOfTrailingZeros. Again this only works for signed numbers. Using our number from earlier, the answer returned is 0. That’s because the number does not end with zeros.
Reversing bits in the Java Integer Class
We can reverse the bits in an Integer using a method called reverse(). This method reverses the bits, but returns an int. If you print it out, you’ll just get the decimal number. If we want to see the bits, we’ll have to convert it to a string like this.
We can also reverse the bytes in a integer using the method reverseBytes(). We might use this method when reading bytes stored in a different order that what Java uses. This method also returns an int. What this method does is reverse our int a byte at a time, not a bit. So when we reverse this number’s bytes, it looks like this. I’m printing it out as hex, because it’s easier to see the reversal.
Shifting bits in Java
If you remember, we could shift bits left and right using the operators shift right (>>) and shift left (<<). These operations are also available on the Integer class. The methods are rotateRight and rotateLeft. Lets start with the number 3. In binary it looks like this (11).
If we pad the missing zeros for the full integer, it looks like this. When we rotate left by two positions, the ones move left.
When we rotate the same number to the right by two positions, we get a big negative number (-1073741824). The reason is the left most bit gets the right most bit. You can think of it as circular. Since the first bit is 1 now, the number becomes negative.
If we just want to know the sign of the number, we can call the method signum(). This returns -1 for negative numbers, 0 for zero, and 1 for positive numbers.
Floats also have a few bit twiddling methods. If we want to create a representation of our float according to the IEEE 754 floating-point bit layout we can call the method floatToIntBits(). This gives us the integer representation of the float as an int. This is great for learning how numbers are stored internally!
Conclusion
And that’s all we’ll cover for the Number class methods. If you have any questions, add them to the comments below. We are done with the Number class and we’re moving on to the Java String class next. If you enjoyed the video, please share and like. You’ll also want to sign up for the DeegeU newsletter. That’s a monthly email containing the month’s videos, plus tips and news not in the videos.
And with that, I’ll see you in the next tutorial!
<!-- DeegeU - Right Side -->
<ins class="adsbygoogle" style="display:inline-block;width:336px;height:280px" data-ad-client="ca-pub-5305511207032009" data-ad-slot="5596823779"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script></p>
Tools Used
- Java
- NetBeans
Media Credits
All media created and owned by DJ Spiess unless listed below.
- No infringement intended
Music:
Talky Beat by Twin Musicom is licensed under a Creative Commons Attribution license (https://creativecommons.org/licenses/by/4.0/)
Source: http://www.twinmusicom.org/song/265/talky-beat
Artist: http://www.twinmusicom.org
Get the code
The source code for “Are you ready to tackle the fizzbuzz test in Java?” can be found on Github. If you have Git installed on your system, you can clone the repository by issuing the following command:
git clone https://github.com/deege/deegeu-java-intro.git
Go to the Support > Getting the Code page for more help.
If you find any errors in the code, feel free to let me know or issue a pull request in Git.
Comments
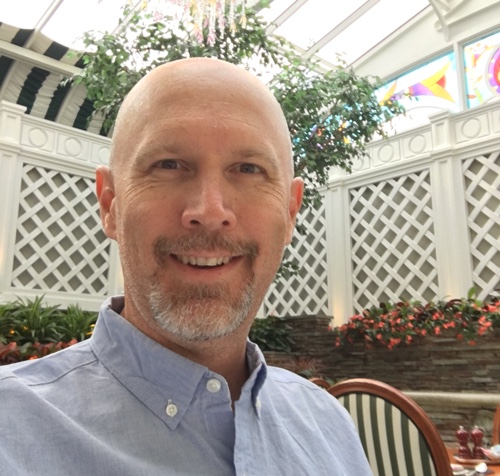
DJ Spiess
Your personal instructor
My name is DJ Spiess and I'm a developer with a Masters degree in Computer Science working in Colorado, USA. I primarily work with Java server applications. I started programming as a kid in the 1980s, and I've programmed professionally since 1996. My main focus are REST APIs, large-scale data, and mobile development. The last six years I've worked on large National Science Foundation projects. You can read more about my development experience on my LinkedIn account.